Custom Salesforce API updates with an Azure Logic App
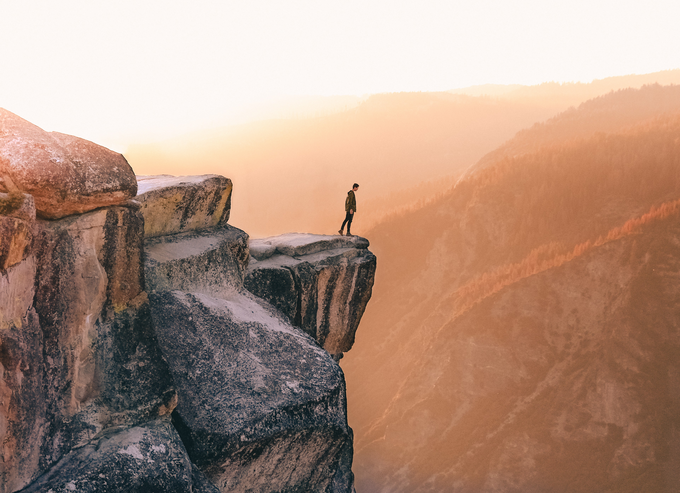
Scenario: You’re using an Azure Logic App to update a Salesforce record using the Salesforce API connector.
Problem: The Salesforce connector doesn’t make it easy to send a custom update request. It wants you to name all the fields you intend to update. But what if you don’t want to update some of those fields, based on some condition? Well, you were up a creek — until you found this post.
Solution: Use the “Parse JSON” Logic App action to build the request you want, then pass this to the body of the Salesforce connector.
Caveat: This solution will essentially break the Logic App designer. You’ll need to use Code View to edit your logic app.
You can file this under “Why in the world would you want to do this?” But hey, you never know. :)
Example
Let’s say you’ve got the following update to send to your Salesforce API:
{
"FirstName": "Light",
"LastName": "Beam",
"Zipcode": "12345"
}
That’s great. You setup your Azure Logic App Salesforce connector, add your three parameters, and the update goes off without a hitch.
But then you want to use the same connector to send the following update request:
{
"Zipcode": "12345"
}
What happens to FirstName
and LastName
? Will the SFDC API spit back an error? Will those fields be set to null
? You don’t want either scenario. No, you want to be able to send the Salesforce API an update request with your own custom content body. Fortunately this is possible using the the Azure Logic App’s very own “Parse JSON” action. All you do is define a JSON schema for your content body, then pass that to the Salesforce Update connector as the body
input.
What we need is an example.
Demo: Building a Logic App to update Salesforce with a custom request body
1. Define your HTTP trigger
First, you’ll need to get your request data from somewhere. An HTTP trigger is a good idea. This also allows you to craft your JSON request somewhere else using a more powerful language like C# or Node, then post that to your Logic App’s HTTP endpoint.
Let’s create an HTTP trigger with a request body JSON schema like this:
{
"properties": {
"value": {
"properties": {
"SomeRecordId": {
"type": "string"
},
"UpdateRequest": {
"type": "string"
}
},
"required": [
"UpdateRequest",
"SomeRecordId"
],
"type": "object"
}
},
"type": "object"
}
That let’s us pass in the record ID to update, as well as the serialized update request.
Next, we need to parse UpdateRequest
.
2. Parse the update request
Why can’t we just pass in @triggerBody()?['value']?['UpdateRequest']
to the Salesforce update request body? Because that would be too easy. :) The Salesforce connector ends up setting your content type to text/plain
instead of application/json
, which doesn’t work. Booo…
So here’s what we’ll do:
- Add a new “Parse JSON” action.
- Set it’s Content to the
UpdateRequest
from the HTTP request’s trigger body. (@triggerBody()?['value']?['UpdateRequest']
if you’re working in Code view.) - Set it’s schema to something like:
{
"properties": {
"FirstName": {
"type": ["string", "null"]
},
"LastName": {
"type": ["string", "null"]
},
"Zipcode": {
"type": ["string", "null"]
},
},
"type": "object"
}
Note the "type": ["string", "null"]
statements. Those are important because they allow those fields to be null
. If they’re null
— and this is key — they won’t be included in the JSON request we send to the Salesforce API. That means those values won’t be updated. Perfect for our demo use case!
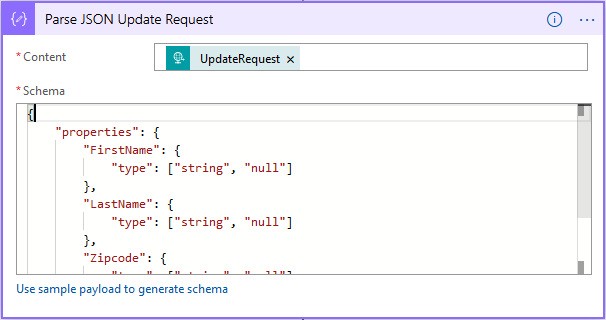
Our last step is to set up our Salesforce connector to use our newly parsed JSON object.
3. Configure the Salesforce Update action
Next we need to tell the Salesforce Update action to use our swanky new JSON object. This will require going to… Code View!
- Switch to Code View
- Find where your Salesforce Update action is defined
- Copy the existing code somewhere safe
- Swap out the existing code with something like this:
"Update_record": {
"inputs": {
"body": "@outputs('Parse_JSON_Update_Request')?['body']",
"host": {
...
},
"method": "patch",
"path": "..."
},
"runAfter": {
"Parse_JSON_Update_Request": [
"Succeeded"
]
},
"type": "ApiConnection"
}
Of course you’ll need to adjust that snippet for your own environment:
- Replace
'Parse_JSON_Update_Request'
with the name of your Parse JSON output. - Use the
host
value from your original Salesforce Update action - Use the
path
value from your original Salesforce Update action
After that, you should be ready to test it out.
4. Test your custom Salesforce updating Logic App
You can use something like Postman to test out your new Logic App.
- In Postman, create a new POST request for your logic app’s public URL
- In the Headers tab, add a new
Content-Type
header set toapplication/json
- In the Body tab, change the selection to “raw”, then paste in some JSON like this:
{
"value": {
"SomeRecordId": "38283298",
"UpdateRequest": {
"Zipcode": "12345",
}
}
}
Note that the fields are nested within a “value” object. That is important for things to be parsed properly.
Of course, you’ll need a real record ID. Real everything, really. Once you’re using data and schema corresponding to your own Salesforce environment, click Send in Postman and watch the world burn. If all goes well, you should have updated your record in Salesforce, leaving FirstName and LastName alone. Yay!
Salesforce and Azure Logic Apps can both be a real pain in the neck. Hopefully this post helped to ease the burden a bit.