Migrate Your DNS Zones from EdgeCast to Azure DNS
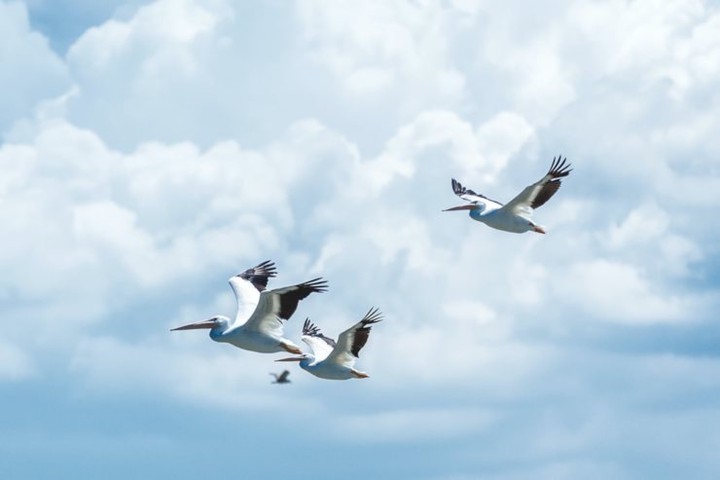
You’ve got a bunch of DNS zones hosted on Verizon’s Edgecast, and you’d like to move them over to Azure DNS. Sounds tedious and error-prone. Let’s use the API’s for Edgecast and Azure to automate copying over those DNS records.
Step 0. Prerequisites
First off, I’ll be using PowerShell 7+, because it’s great, and it’s platform independent. (Get PowerShell )
Step 1. Setup your Edgecast API
When you visit your Edgecast dashboard , there’s no clue that an awesome API is lurking beneath. There is only lots of manual drudgery. Fortunately there is a pretty comprehensive REST API document . If you like Docs, that’s all you’ll need for this section.
You’ll need two pieces of information to use the API
- Your account number, visible in parentheses next to your account name in the upper right hand corner of the Edgecast dashboard.
- Your API token, accessible from the settings page.
Now let’s try it out!
$edgeCastToken = "_YOUR_TOKEN_HERE"
$edgeCastCustId = "_YOUR_ID_HERE_"
Invoke-RestMethod -SkipHeaderValidation -Headers @{ 'Authorization'= "tok:$edgecasttoken"} -Uri https://api.edgecast.com/v2/mcc/customers/$edgeCastCustId/dns/routezones
(Note that we have to use the -SkipHeaderValidation
option because “tok:” is a nonstandard header value)
Step 2. Setup the Azure Az PowerShell module
First make sure the Az module is installed . This is how you’ll work with your Azure subscription(s) from PowerShell.
Basically, you’ll run this:
if ($PSVersionTable.PSEdition -eq 'Desktop' -and (Get-Module -Name AzureRM -ListAvailable)) {
Write-Warning -Message ('Az module not installed. Having both the AzureRM and ' +
'Az modules installed at the same time is not supported.')
} else {
Install-Module -Name Az -AllowClobber -Scope AllUsers
}
Unfortunately, it tends to be really slow. You’ll need to say “Y” or “A” when it asks if you’re sure you want to install.
Step 3. Authenticate with Azure
To authenticate with Azure, run Connect-AzAccount
and follow the directions.
When you have multiple subscriptions
If you manage multiple Azure subscriptions, you’ll need to change the active Azure context.
Grab the subscription ID of the subscription you want to manage by running Get-AzSubscription
. 👈 That also lets you know if you successfully connected to Azure account. :)
$context = Get-AzSubscription -SubscriptionId _SUBSCRIPTION_GUID_
Set-AzContext $context
Step 4. Grab all your Edgecast DNS zones
First, let’s streamline Edgecast API access with a Powershell function:
function Invoke-EdgecastApi($Uri) {
try {
$result = Invoke-RestMethod -SkipHeaderValidation -Headers @{ 'Authorization'= "tok:$EdgeCastToken"} -Uri $Uri
return $result
}
catch {
Write-Verbose $_
}
return $null
}
With that out of the way, grabbing your Edgecast DNS zones is as easy as:
$edgecastZones = Invoke-EdgecastApi -Uri https://api.edgecast.com/v2/mcc/customers/$EdgecastCustomerId/dns/routezones
$edgecastZones | ForEach-Object { Write-Line $_.DomainName }
Step 5. Grab an Edgecast zone’s record information
Let’s start with the first zone from the collection of zones we retrieved in the previous step. This is how you would get its detailed zone information, including the all-important DNS records.
$edgecastZone = $edgecastZones | Select -First 1
$edgecastZoneInfo = Invoke-EdgecastApi -Uri https://api.edgecast.com/v2/mcc/customers/$EdgecastCustomerId/dns/routezone?id=$($edgecastZone.ZoneId)
The DNS records will be inside $edgeZoneInfo.Records
.
Step 6. Create the zone in Azure DNS
$domainName = $edgecastZoneInfo.DomainName.Trim(".")
$AzureResourceGroupName = "MyResourceGroupName"
New-AzDnsZone -Name $domainName -ResourceGroupName $AzureResourceGroupName
Step 7. Migrate the records to Azure DNS
Let’s take the A records for example. Inside $edgeZoneInfo.Records.A
, you’ll see that each A record has three fields, Name
, Rdata
, and TTL
. You just have to create a new DNS A record in Azure DNS for each of the A records you got from Edgecast. Here’s an example, assuming that your DNS record is in a variable called $r
:
New-AzDnsRecordSet -Overwrite -Name $edgecastZone.Name -RecordType A -ZoneName $edgecastZone.Name -ResourceGroupName $AzureResourceGroupName -Ttl $r.TTL -DnsRecords (New-AzDnsRecordConfig -Ipv4Address $r.Rdata)
Step 8. Automate!
Once you’ve kicked the tires, it’s time to automate. I whipped up a script and stuck it in a repo on GitHub called Edgecast2AzureDns .
Go fork and conquer!