Display a QR code on the fly from zsh
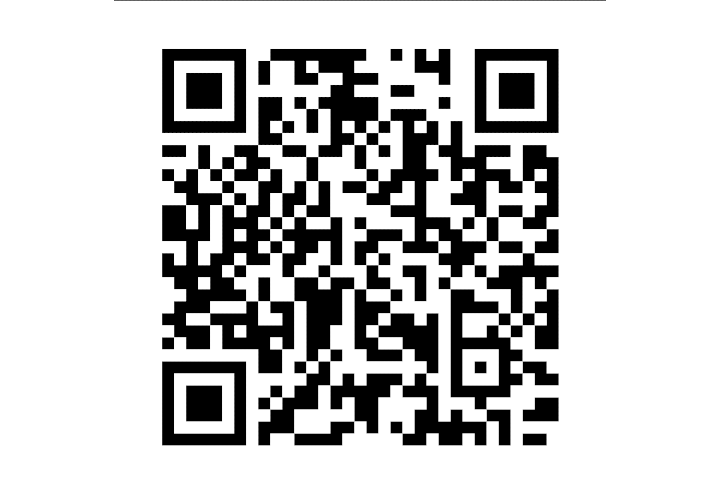
You’re typing away at the console in Linux or Mac OS when you suddenly need to display a QR code. There are a lot of dubious websites that purport to generate QR codes for you, but at what cost?
Let’s just generate our own QR codes using open-source tools.
1. Install prerequisites
First, we need to install a few packages.
- qrencode : OSS utility for generating QR codes
- feh : Lightweight OSS X11 image viewer
- xclip
: Access the clipboard if you want to generate a QR code from the contents of the clipboard. Only necessary on Linux because Mac has
pbpaste
built in, which does the same thing.
Linux
If using apt
, installation would look something like:
sudo apt install qrencode feh xclip
Mac OS
If you use Homebrew:
brew install qrencode
brew install feh
2. Write a little zsh script
#!/usr/bin/env zsh
# Make sure we have all the utilities we need
requiredBins=('qrencode' 'feh')
if [[ `uname` == "Darwin" ]]; then
requiredBins+=("pbpaste")
else
requiredBins+=("xclip")
fi
missingBins=()
for cmd in $requiredBins
do
if ! which $cmd > /dev/null; then
missingBins+=($cmd)
fi
done
if [ ${#missingBins[@]} -gt 0 ]; then
IFS=","
echo "OOPS! Missing $missingBins"
exit 1
fi
# Clipboard paste function which works on Mac and Linux
paste() {
if which pbpaste > /dev/null; then
pbpaste
else
xclip -selection clipboard -o
fi
}
# Try using the command line argument as the QR source text
sourceText=$1
if (( # == 0 )); then
# No argument. Grab from clipboard instead
sourceText=$(paste)
fi
# Convert the text to a QR code and display it with feh
echo $sourceText | qrencode --size=15 -o - | feh -
The same script is on GitHub , in case that’s hard to read up there.
3. Execute your script
- Save your new script somewhere like
~/.local/bin/qr
- Make it executable:
chmod +x ~/.local/bin/qr
- Run it:
qr 'some text'
- Or copy some text to the clipboard and run it with
qr
4. Enjoy your nice new QR code
Ahhh. Now that’s a nice QR code. 😎